Operators in spEL
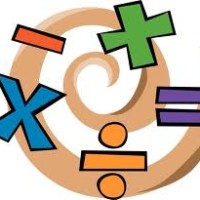
![]() |
|
The various operators that are most frequently used in this context are :-
Relational operators
- equal (==)
- not equal (!=)
- less than (<)
- less than or equal (<=)
- greater than (>)
- greater than or equal (>=)
Logical operators
- And(&&)
- Or(||)
- not (!).
Mathematical operators
- addition (+)
- Subtraction (-)
- Multiplication (*)
- division (/)
- modulus (%)
- exponential power (^).
Ternary operator
- if-then-else (<condn> ? <stmt1 if true> : <Stmt2 if false>)
.
- if-then-else (<condn> ? <stmt1 if true> : <Stmt2 if false>)
Step 1 : Create a sample POJO
File : Operators.java
package com.simpleCodeStuffs.spEL; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; @Component("operators") public class Operators { @Value("#{1==1}") private boolean equals; public boolean isEquals() { return equals; } public void setEquals(boolean equals) { this.equals = equals; } }
Step 2 : Create the main class
File : MainClass.java
package com.simpleCodeStuffs.spEL; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("elBeans.xml"); Operators op = (Operators) context.getBean("operators"); System.out.println("equals" + op.isEquals()); } }
Step 3 : Enable automatic component scanning in elBeans.xml
File : elBeans.xml
Step 4 : Run the program. The output is
Step 5 : Other operators and the functionalities
@Value("#{1 != 5}") //true private boolean NotEqual; @Value("#{1 < 1}") //false private boolean LessThan; @Value("#{1 <= 1}") //true private boolean LessThanOrEqual; @Value("#{1 > 1}") //false private boolean GreaterThan; @Value("#{1 >= 1}") //true private boolean GreaterThanOrEqual; /* * Logical operators , numberBean.no == 999 * @Value("#{numberBean.no == 999 and numberBean.no < 900}") * can be used in case 'numberBean' is another bean. */ @Value("#{999 == 999 and 999 < 900}") //false private boolean And; @Value("#{999 == 999 or 999 < 900}") //true private boolean Or; @Value("#{!(999 == 999)}") //false private boolean Not; //Mathematical operators @Value("#{1 + 1}") //2.0 private double testAdd; @Value("#{'1' + '@' + '1'}") //1@1 private String AddString; @Value("#{1 - 1}") //0.0 private double Subtraction; @Value("#{1 * 1}") //1.0 private double Multiplication; @Value("#{10 / 2}") //5.0 private double Division; @Value("#{10 % 10}") //0.0 private double Modulus ; @Value("#{2 ^ 2}") //4.0 private double ExponentialPower;
//Ternary operator @Value("#{99!=0?'expresion holds true':'expression is false'}") private String name; public String getName() { return name; } public void setName(String name) { this.name = name; }
File : Operators.java
package com.simpleCodeStuffs.spEL; import org.springframework.beans.factory.annotation.Value; import org.springframework.stereotype.Component; @Component("operators") public class Operators { @Value("#{99!=0?'expresion holds true':'expression is false'}") private String name; public String getName() { return name; } public void setName(String name) { this.name = name; } }
File : MainClass.java
package com.simpleCodeStuffs.spEL; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class MainClass { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("elBeans.xml"); Operators op = (Operators) context.getBean("operators"); System.out.println(op.getName()); } }
The output is -
![]() |
|